Build an HTTP API
An HTTP API is a way for programs to communicate over the internet using HTTP. HTTP is the same protocol used by your web browser, but APIs are built to be used by programs rather than people. py.space makes it easy to build HTTP APIs – let’s see how.
Example: What’s the time?
Did you know that all GPS satellites do is broadcast the current time? Their atomic clocks are so precise that a GPS receiver can receive timestamps from three satellites, measure the tiny differences between them, and work out how far away they are using the speed of light. 🤯
Well, we don’t have an atomic clock, but we can make an API that returns the current time! Here’s an example API that does just that:
Here’s how you build it:
1. Create an HTTP API widget
Go to your account’s homepage, and under “What do you want to build?”, click HTTP API.
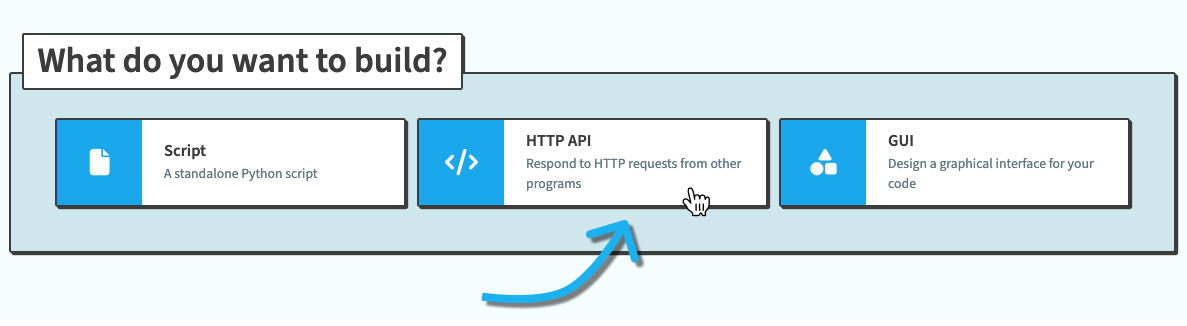
By default, the widget will already have an HTTP API set up. We’ll modify this in the next step
2. Get the time
Let’s modify the default code so that when we hit the HTTP endpoint, our function returns the current time.
First, we need to import datetime
at the top of our code. Add it under import anvil.server
:
import anvil.server
from datetime import datetime
By default, our widget already has a URL set up, and the function in our code has the @anvil.server.route()
decorator. This means we can make HTTP requests to our widget by accessing our widget’s URL and the path passed into @anvil.server.route()
. Let’s change the path from /hello
to /time
:
@anvil.server.route('/time')
Finally, we can change the function so that it returns the current time as a dictionary, which py.space will automatically turn into JSON when the HTTP endpoint is hit. We’ll use datetime.now()
to get the current time:
def current_time(**p):
print(f"The time is {datetime.now()}")
return {"time": datetime.now().isoformat()}
3. Try it out
In the bottom-right of your widget, you’ll see the URL of your function – in our example above, it’s https://bony-spectacular-season.anvil.app/time
.
If you’re using Linux or MacOS, you can open a terminal and request that URL with curl
. Here’s how that looks for me:
$ curl https://bony-spectacular-season.anvil.app/time
{"time":"2024-05-03T20:46:04.397923"}
Because this is a simple “GET” endpoint, you could also just copy-paste the URL into your browser!
4. See the output
If you look at your widget, you’ll see that your code also print()
ed the current time, and that output showed up in the Output panel.
When you’re building an HTTP API, it can get tricky to work out what’s going on when you receive a request. In py.space, you can use print
statements in your HTTP endpoints to see your debugging output in the Output panel.
5. Share it!
Click the the Public button at the top of the widget to make it public on your py.space profile!
What next?
There’s more to explore out in py.space! Why not check out another tutorial?
- Create a simple script that fetches weather data from an API
- Build a GUI widget to keep a daily log
- Create a web scraper that runs as a scheduled task
Do you still have questions?
Our Community Forum is full of helpful information and friendly experts.