Build a Scheduled Task
Scheduled tasks, also known as scheduled jobs or cron jobs, are automated processes executed at specific times or intervals. They’re great for repetitive operations, like backing up data, sending reports, or updating website content.
In py.space, scheduled tasks are just script widgets that have been scheduled to run at regular intervals.
To create a scheduled task, all you need to do is create a script widget, press the “Schedule” button and choose when you want your code to run!
In this tutorial, you’ll use the BeautifulSoup
library to build a web scraper that runs every day and emails you a quote of the day.
Example: Quote Web Scraper
Web scrapers extracts information from websites. In this tutorial, we’ll build a simple web scraper to extract the “Quote of the Day” from the Wikiquote page. We’ll schedule our script to run every day and email us the quote!
Here’s how you build it:
1. Create a Script widget
Go to your account’s homepage, and under “What do you want to build?”, click Script.
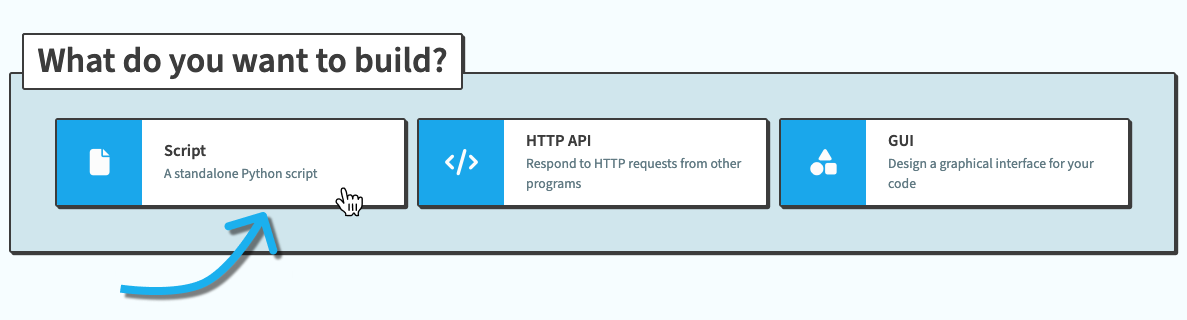
By default, the script will come with a little “Hello World” content. Delete that code since we won’t be using it.
2. Install and import packages
The first thing we need to do is install the BeautifulSoup
library. Click on the “Packages” tab at the top right. Where it says “Add package…”, type in beautifulsoup4
, leave the version tag empty and click “Add”.
Now we can import all the packages we need at the top of our script. Besides BeautifulSoup
, we’ll also be using the Python requests
package and the Anvil email service.
import requests
import anvil.email
from bs4 import BeautifulSoup
3. Build the web scraper
We’re now ready to build the web scaper. First, we’ll use requests
to get the “Quote of the Day” page from WikiQuote then create a BeautifulSoup object and pass in the content of that page:
response = requests.get("https://en.wikiquote.org/wiki/Wikiquote:Quote_of_the_day")
soup = BeautifulSoup(response.text, "html.parser")
Next, we’ll use BeautifulSoup to find the quote of the day from the webpage:
main_table_body = soup.find('tbody')
cells = main_table_body.find('tbody').find_all('td')
for br in cells[0].find_all("br"):
br.replace_with("\n")
quote = cells[0].text.strip()
author = cells[1].text.strip()
Finally, we can send ourselves an email with the quote of the day!
text = f"Here is today's quote of the day:\n\n{quote}\n\n{author}"
anvil.email.send(
from_name = "Quote of the Day",
subject = "Today's Quote",
text = text
)
4. Set up a Scheduled Task
Let’s now turn our script into a scheduled task and set it to run every day! At the bottom-right of the widget editor, click Schedule. In the pop-up box, choose when you want to run this task:
“Every 1 day at 08:00 UTC” should do the trick:
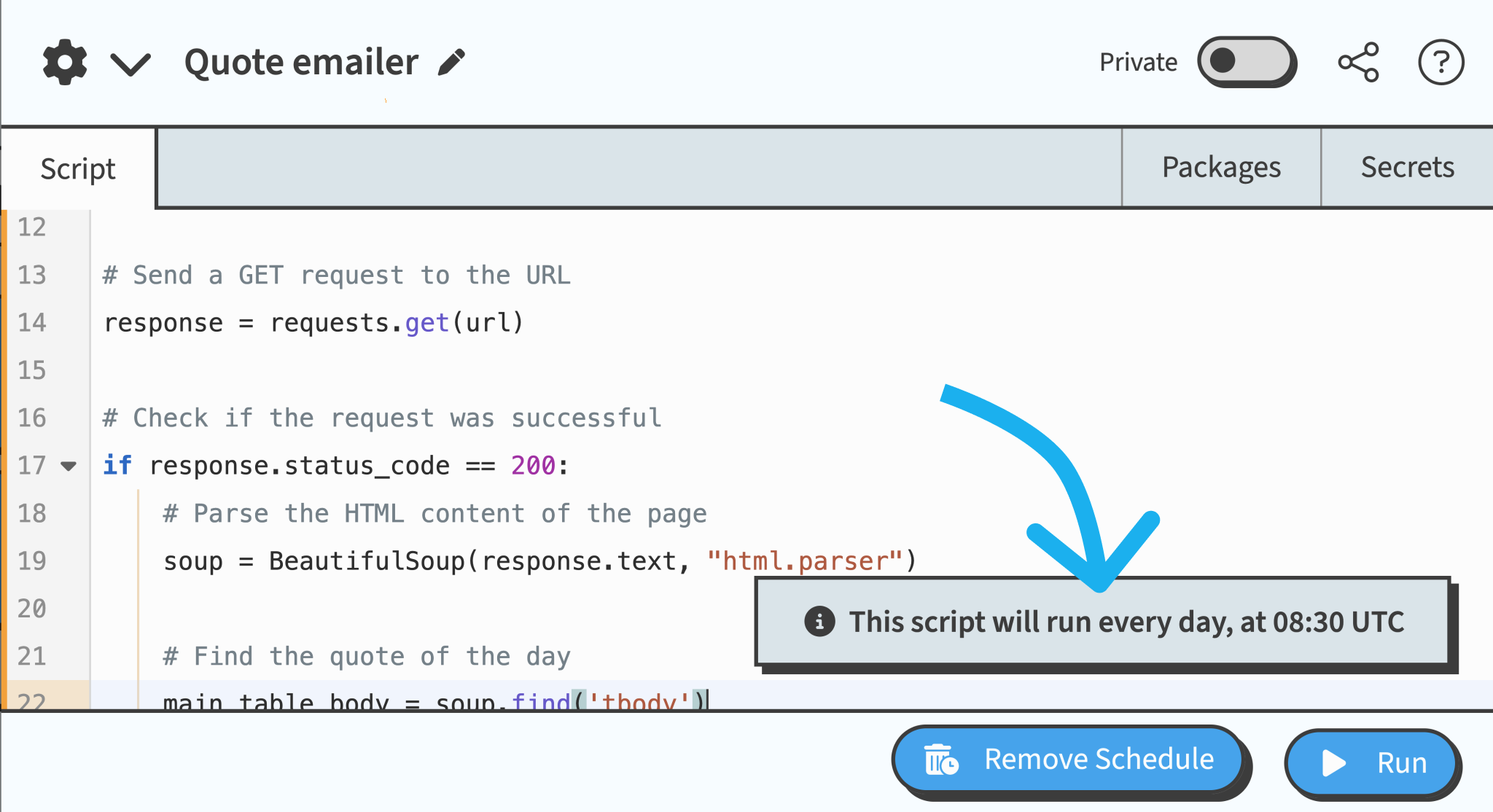
Schedule Dialog Box
5. Run it, just to be sure
You can test your Scheduled Task immediately by clicking Run. If you wait a few moments and check the email you used to sign up for py.space, you’ll see you now have a message.
You will now receive the WikiQuote Quote of the Day by email every day!
6. Share it!
Click the Public button at the top of the widget to make it public on your py.space profile!
What next?
There’s more to explore out in py.space! Why not check out another tutorial?
- Create a simple script that fetches weather data from an API
- Build a GUI widget to keep a daily log
- Create an HTTP API that returns the current time
Do you still have questions?
Our Community Forum is full of helpful information and friendly experts.