Build a Script
py.space allows you to build and run simple python scripts on the web. You can easily install any Python packages you need and run your script without needing to set up an environment. Because py.space scripts run on the web and are easy to set up, they are the perfect place to fetch data from web APIs.
This quick tutorial will show you how to create a py.space script that uses the requests
library to fetch and print data from a weather API.
Example: Which City is Warmer?
This py.space script uses an API to fetch the current temperature of three different cities and tells you which one is warmer:
Here’s how you build it:
1. Create a Script widget
Go to your py.space dashboard, and under “What do you want to build?”, click Script.
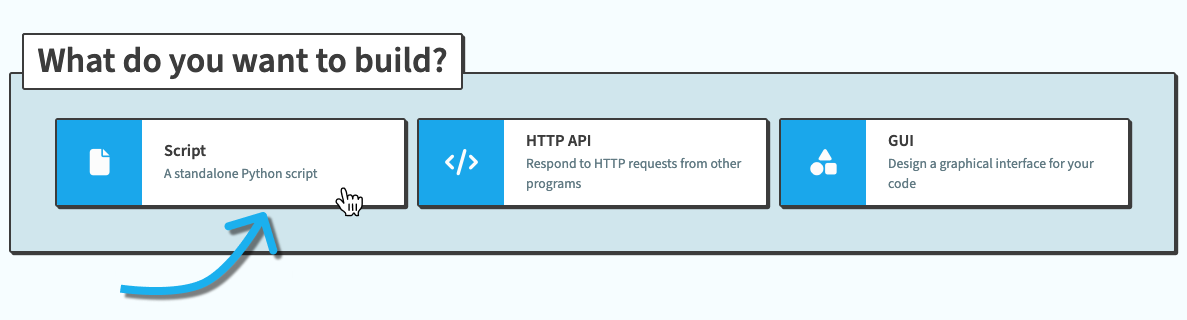
By default, the script will come with a little “Hello World” content. Delete that code since we won’t be using it.
2. Add a Secret
Our script is going to use a weather API to fetch weather data and for that, we need an API key. You can get one for free at weatherapi.com.
We now need to add the API key as a secret in our py.space widget.
Click Secrets in the top-right of the widget editor, then choose Create a new secret. Call it api_key
, then click the Edit button to set the value of the secret. Paste in your own API key.
3. Call the API
Let’s now write some code. We need to use the requests
package and our API key in order to fetch the weather data so add the following import statements to the top of your script:
from anvil.secrets import get_secret
import requests
Let’s now add a function that takes in a city name and gives us the current weather:
def get_temp(city):
return requests.get(f"https://api.weatherapi.com/v1/current.json?key={get_secret('api_key')}&q={city}&aqi=no").json()['current']['temp_c']
Next, we need a list of cities. We can then create a dictionary of temperatures by passing each city into the get_temp
function.:
cities = ["London", "Madrid", "Chicago"]
temps = { city: get_temp(city) for city in cities }
The temps
dictionary will look something like this: {'London': 11.0, 'Madrid': 18.0, 'Chicago': 15.6}
To find the warmest city, all we need to do is find which value in our dictionary is the largest and print out its key:
warmest = max(temps, key=temps.get)
print(f"The warmest city is {warmest}!")
4. Run it
Click Run in the bottom right of the widget to run your script and see which city is the warmest!
5. Share it!
Click the Public button at the top of the widget to make it public on your py.space profile!
Because you’ve stored your API key in your widget’s secrets, you can share your code with the world and still keep your API key secret.
What next?
There’s more to explore out in py.space! Why not check out another tutorial?
- Create a web scraper that runs as a scheduled task
- Build a GUI widget to keep a daily log
- Create an HTTP API that returns the current time
Do you still have questions?
Our Community Forum is full of helpful information and friendly experts.